智能助手 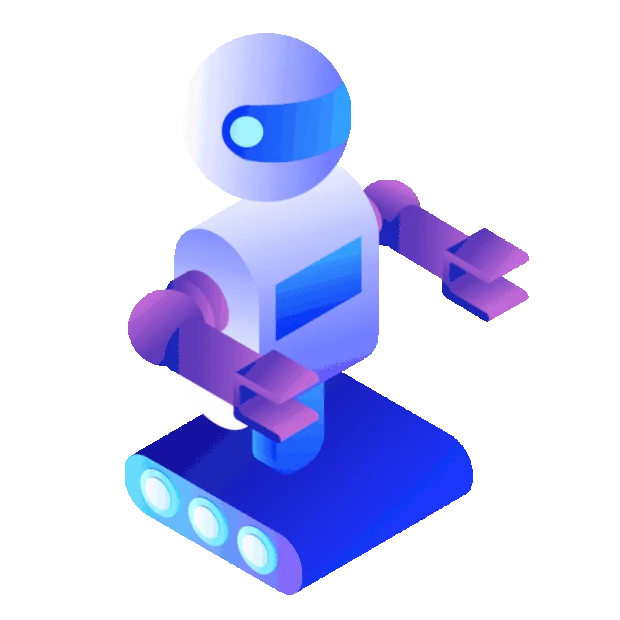
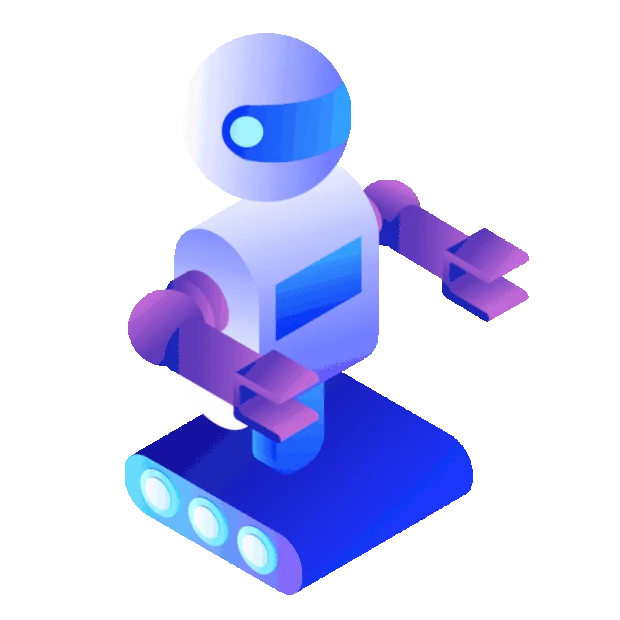
外观
约 1251 字大约 4 分钟
2025-02-20
在前端开发中,捕获错误是保证应用稳定性和用户体验的关键。为了全面捕获前端的错误,我们需要覆盖多种错误类型,并采用多种捕获机制。以下是全面捕获前端错误的策略和实现方法。
前端错误主要分为以下几类:
window.onerror = function (message, source, lineno, colno, error) {
console.error('全局错误:', { message, source, lineno, colno, error });
// 将错误信息上报到服务器
reportErrorToServer({ message, source, lineno, colno, error });
return true; // 阻止默认错误处理
};
window.addEventListener('error', function (event) {
if (event.target && (event.target.tagName === 'IMG' || event.target.tagName === 'SCRIPT' || event.target.tagName === 'LINK')) {
console.error('资源加载错误:', event.target.src || event.target.href);
// 将错误信息上报到服务器
reportErrorToServer({ type: 'resource', url: event.target.src || event.target.href });
}
}, true); // 使用捕获模式
window.onunhandledrejection = function (event) {
console.error('未捕获的 Promise 错误:', event.reason);
// 将错误信息上报到服务器
reportErrorToServer({ type: 'promise', reason: event.reason });
};
Vue 2:
Vue.config.errorHandler = function (err, vm, info) {
console.error('Vue 错误:', err, info);
// 将错误信息上报到服务器
reportErrorToServer({ type: 'vue', err, info });
};
React:
class ErrorBoundary extends React.Component {
componentDidCatch(error, info) {
console.error('React 错误:', error, info);
// 将错误信息上报到服务器
reportErrorToServer({ type: 'react', error, info });
}
render() {
return this.props.children;
}
}
// 使用 ErrorBoundary 包裹组件
<ErrorBoundary>
<MyComponent />
</ErrorBoundary>
function runAsyncCode(callback) {
try {
callback();
} catch (error) {
console.error('异步代码错误:', error);
// 将错误信息上报到服务器
reportErrorToServer({ type: 'async', error });
}
}
setTimeout(() => runAsyncCode(() => {
throw new Error('异步错误');
}), 1000);
使用 try-catch 包裹跨域脚本的执行。
在跨域脚本中手动捕获错误并通知主页面。
捕获错误后,需要将错误信息上报到服务器,以便分析和修复。常见的上报方式包括:
function reportErrorToServer(error) {
const url = 'https://your-server.com/log-error';
const data = JSON.stringify(error);
fetch(url, {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: data,
}).catch((err) => {
console.error('上报错误失败:', err);
});
}
Sentry:https://sentry.io/
Bugsnag:https://www.bugsnag.com/
LogRocket:https://logrocket.com/
这些服务提供了完整的错误监控和上报功能,可以快速集成到项目中。
以下是一个完整的错误捕获和上报示例:
// 全局错误捕获
window.onerror = function (message, source, lineno, colno, error) {
console.error('全局错误:', { message, source, lineno, colno, error });
reportErrorToServer({ type: 'global', message, source, lineno, colno, error });
return true;
};
// 资源加载错误捕获
window.addEventListener('error', function (event) {
if (event.target && (event.target.tagName === 'IMG' || event.target.tagName === 'SCRIPT' || event.target.tagName === 'LINK')) {
console.error('资源加载错误:', event.target.src || event.target.href);
reportErrorToServer({ type: 'resource', url: event.target.src || event.target.href });
}
}, true);
// Promise 未捕获错误
window.onunhandledrejection = function (event) {
console.error('未捕获的 Promise 错误:', event.reason);
reportErrorToServer({ type: 'promise', reason: event.reason });
};
// Vue 错误捕获
Vue.config.errorHandler = function (err, vm, info) {
console.error('Vue 错误:', err, info);
reportErrorToServer({ type: 'vue', err, info });
};
// 上报错误到服务器
function reportErrorToServer(error) {
const url = 'https://your-server.com/log-error';
const data = JSON.stringify(error);
fetch(url, {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: data,
}).catch((err) => {
console.error('上报错误失败:', err);
});
}
通过全局错误捕获、资源加载错误捕获、Promise 错误捕获、框架错误捕获等多种机制,可以全面捕获前端的错误。捕获错误后,及时上报并分析错误日志,是保证应用稳定性和用户体验的关键。希望这个指南能帮助你更好地处理前端错误!
版权归属:tuyongtao1