智能助手 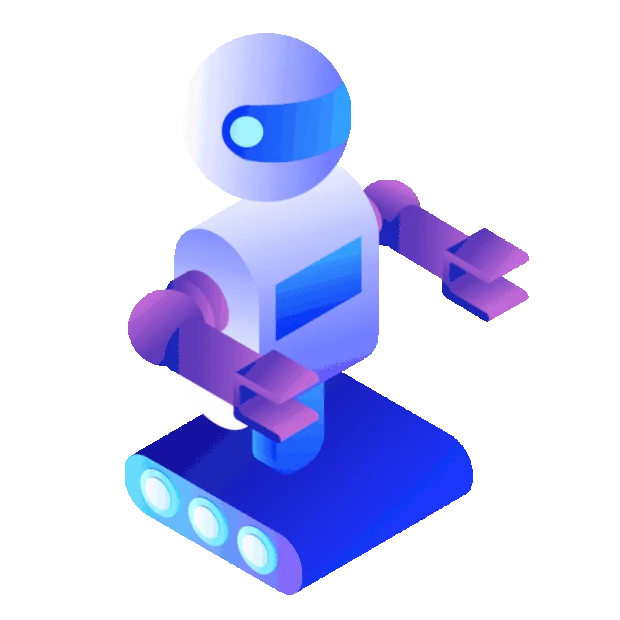
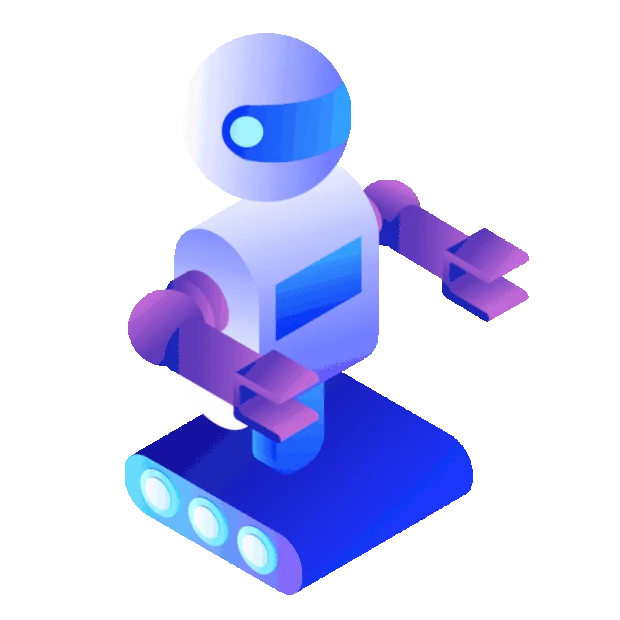
外观
以下案例中的的脚手架已经发布 在 npm , 在本地可直接使用
npm create vue-custom@latest
pnpm create vue-custom@latest
yarn create vue-custom@latest
该案例用最短的代码帮助你理解一个脚手架。
mkdir create-vue-custom
cd create-vue-custom
touch index.js
mkdir scripts
cd scripts
touch build.mjs
pnpm init
此时文件结构应是如下:
- scripts
- build.mjs
- index.js
- package.json
pnpm add esbuild
pnpm add kleur
pnpm add prompts
pnpm add zx
#!/usr/bin/env node
// 上面这行表示 这是一个可执行脚本,执行环境为 node
import prompts from 'prompts' // 交互提示库
import { exec, spawnSync } from 'node:child_process' // node 子进程相关方法
import { red, green, bold } from 'kleur/colors' // 字体美化
const log = console.log
// 定义问题
const questions = [
{
type: 'text',
name: 'projectName',
message: '请输入工程名称',
},
{
type: 'confirm',
name: 'isTemplate',
message: '是否使用内置模板',
initial: true,
},
{
type: (prev) => (prev ? 'select' : null),
name: 'templateType',
message: '请选择模板类型',
choices: [
{ title: 'PC', value: 'pc', description: '这是一个默认的后台管理系统模板' },
{ title: 'H5', value: 'h5', description: '这是一个默认的H5系统模板' },
],
initial: 0,
},
]
// 这是个人的模板
const templateRepo = {
pc: 'https://github.com/tuyongtao-T/vue3-admin-ts.git',
}
async function init() {
try {
log(green('准备执行脚手架命令...'))
// 获取交互内容
const result = await prompts(questions)
// 如果采用模板就从远承拉取
if (result.isTemplate) {
log(green('准备拉取远程模板...'))
// 使用系统拉取命令(这里也可以使用 download-git-repo等拉取git相关的工具)
exec(`git clone ${templateRepo[result.templateType]} ${result.projectName}`)
} else {
// 如果不使用脚手架提供的模板则使用 create-vue脚手架
log(green('准备切换到create-vue...'))
const userAgent = process.env.npm_config_user_agent ?? ''
const packageManager = /pnpm/.test(userAgent)
? 'pnpm'
: /yarn/.test(userAgent)
? 'yarn'
: /bun/.test(userAgent)
? 'bun'
: 'npm'
// 执行create-vue脚手架命令
spawnSync(`${packageManager}`, ['create', 'vue@latest', result.projectName], { stdio: 'inherit' })
}
} catch (error) {
console.log(error)
}
}
init().catch((error) => {
log(bold(error))
process.exit(1)
}).finally(() => {
log(green('创建成功...'))
})
这里使用 esbuild 进行构建。 在 scripts/build.cjs 中添加内容:
import * as esbuild from "esbuild";
await esbuild.build({
bundle: true,
entryPoints: ["index.js"],
outfile: "outfile.cjs",
format: "cjs",
platform: "node",
target: "node14",
});
"name": "create-vue-custom",
"version": "0.0.1",
"type": "module",
"bin": {
"custom-cli": "outfile.cjs"
},
"files": [
"outfile.cjs"
],
"scripts": {
"build": "zx ./scripts/build.mjs"
},
pnpm build
npm login
npm publish
至此你已经把你的脚手架发布在 npm 上了, 可让其他人来下载使用。 git 仓库
Prompts.js(https://chinabigpan.github.io/prompts_docs_cn/)
Inquirer.js
command.js :交互+参数解析
parseArgs(node:util):node17+
minimist
arg
command.js :交互+参数解析
版权归属:tuyongtao1