智能助手 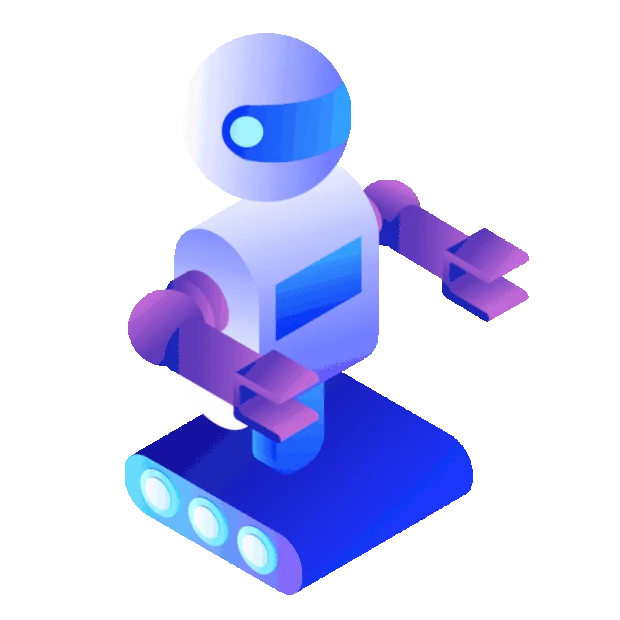
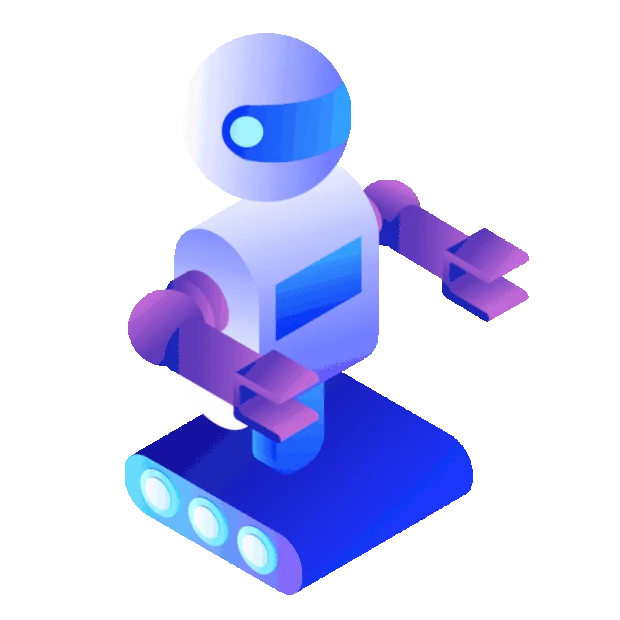
外观
约 1215 字大约 4 分钟
2024-07-20
复杂度 时间复杂度: O(n)
空间复杂度: O(1)
Code
/**
* @param {number[]} numS
* @param {number} target
* @return {number[]}
*/
var twoSum = function (numS, target) {
let obj = {}
let len = numS.length
for (let i = 0; i < len; i++) {
let temp = target - numS[i]
if (Reflect.has(obj, temp)) {
console.log([i, obj[temp]])
return [i, obj[temp]]
} else {
obj[numS[i]] = i
}
}
}
字母异位词 是由重新排列源单词的所有字母得到的一个新单词。
const groupAnagrams = function(strs) {
const obj = {}
for (const iterator of strs) {
let arr = Array.from(iterator)
let temp = arr.sort().toString()
if(Reflect.has(obj,temp)) {
obj[temp].push(iterator)
}else {
obj[temp] = [iterator]
}
}
return Object.values(obj)
};
const longestConsecutive = function(nums) {
let set = new Set(nums)
let long = 0
for (const iterator of set) {
if(!set.has(iterator-1)) {
let curNum = iterator
let curLong = 1
while(set.has(curNum+1)) {
curNum +=1
curLong +=1
}
long = Math.max(long, curLong)
}
}
return long
};
const moveZeroes = function (nums) {
const removeEl = (nums, val) => {
let fast = 0
let slow = 0
let len = nums.length
for (; fast < len; fast++) {
if (nums[fast] !== val) {
nums[slow] = nums[fast]
slow++
}
}
return slow
}
let slowIndex = removeEl(nums, 0)
for (let i = slowIndex; i < nums.length; i++) {
nums[i] = 0
}
return nums
}
找出其中的两条线,使得它们与 x 轴共同构成的容器可以容纳最多的水。
返回容器可以储存的最大水量。
const maxAreaFn = function (height) {
let len = height.length
let first = 0,
end = len - 1
let maxArea = 0
while (first < end) {
let area = Math.min(height[first], height[end]) * (end - first)
maxArea = Math.max(maxArea, area)
if (height[first] < height[end]) {
first++
} else {
end--
}
}
return maxArea
}
const threeSum = function (nums) {
let res = []
let len = nums.length
if (!nums || len < 3) {
return res
}
nums.sort((a, b) => a - b)
for (let i = 0; i < len; i++) {
if (nums[i] > 0) {
break
}
if (i > 0 && nums[i] == nums[i - 1]) continue
let left = i + 1
let right = len - 1
while (left < right) {
const sum = nums[left] + nums[right] + nums[i]
if (sum === 0) {
res.push([nums[i], nums[left], nums[right]])
while (nums[left] === nums[left + 1]) {
left++
}
while (nums[right] === nums[right - 1]) {
right--
}
left++
right--
} else if (sum < 0) {
left++
} else if (sum > 0) {
right--
}
}
}
return res
}
const trap = function (height) {
let len = height.length
let left = 0
let right = len - 1
let sum = 0
let leftMax = 0
let rightMax = 0
while (left < right) {
leftMax = Math.max(leftMax, height[left])
rightMax = Math.max(rightMax, height[right])
if (height[left] < height[right]) {
sum += leftMax - height[left]
left++
} else {
sum += rightMax - height[right]
right--
}
}
return sum
}
给定一个排序数组和一个目标值,在数组中找到目标值,并返回其索引。如果目标值不存在于数组中,返回它将会被按顺序插入的位置。
请必须使用时间复杂度为 O(log n) 的算法。
const searchInsert = function (nums, target) {
let len = nums.length
let left = 0
let right = len - 1
let index = len
while (left <= right) {
let mid = left + Math.floor((right - left) / 2)
if (target <= nums[mid]) {
index = mid
right = mid - 1
} else {
left = mid + 1
}
}
console.log(index)
return index
}
给你一个满足下述两条属性的 m x n 整数矩阵:
每行中的整数从左到右按非严格递增顺序排列。 每行的第一个整数大于前一行的最后一个整数。 给你一个整数 target ,如果 target 在矩阵中,返回 true ;否则,返回 false 。
const searchMatrix = function (matrix, target) {
const m = matrix.length
const n = matrix[0].length
let left = 0
let right = m * n - 1
while (left <= right) {
let mid = left + Math.floor((right - left) / 2)
let value = matrix[Math.floor(mid / n)][mid % n]
if (target < value) {
right = mid - 1
} else if (target > value) {
left = mid + 1
} else {
return true
}
}
return false
}
如果数组中不存在目标值 target,返回 [-1, -1]。
你必须设计并实现时间复杂度为 O(log n) 的算法解决此问题。
版权归属:tuyongtao1